Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples.
A method is a block of code which only runs when it is called.
You can pass data, known as parameters, into a method.
Methods are used to perform certain actions, and they are also known as functions .
Why use methods? To reuse code: define the code once, and use it many times.

Create a Method
A method must be declared within a class. It is defined with the name of the method, followed by parentheses () . Java provides some pre-defined methods, such as System.out.println() , but you can also create your own methods to perform certain actions:
Create a method inside Main:
Example Explained
- myMethod() is the name of the method
- static means that the method belongs to the Main class and not an object of the Main class. You will learn more about objects and how to access methods through objects later in this tutorial.
- void means that this method does not have a return value. You will learn more about return values later in this chapter
Call a Method
To call a method in Java, write the method's name followed by two parentheses () and a semicolon ;
In the following example, myMethod() is used to print a text (the action), when it is called:
Inside main , call the myMethod() method:
Try it Yourself »
A method can also be called multiple times:
In the next chapter, Method Parameters , you will learn how to pass data (parameters) into a method.
Test Yourself With Exercises
Insert the missing part to call myMethod from main .
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
- Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
- Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
- Java Operator Precedence
- Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
Java Math sqrt()
Java Math acos()
Java Math atan()
Java Math tan()
A method is a block of code that performs a specific task.
Suppose you need to create a program to create a circle and color it. You can create two methods to solve this problem:
- a method to draw the circle
- a method to color the circle
Dividing a complex problem into smaller chunks makes your program easy to understand and reusable.
In Java, there are two types of methods:
- User-defined Methods : We can create our own method based on our requirements.
- Standard Library Methods : These are built-in methods in Java that are available to use.
Let's first learn about user-defined methods.
- Declaring a Java Method
The syntax to declare a method is:
- returnType - It specifies what type of value a method returns For example if a method has an int return type then it returns an integer value. If the method does not return a value, its return type is void .
- methodName - It is an identifier that is used to refer to the particular method in a program.
- method body - It includes the programming statements that are used to perform some tasks. The method body is enclosed inside the curly braces { } .
For example,
In the above example, the name of the method is adddNumbers() . And, the return type is int . We will learn more about return types later in this tutorial.
This is the simple syntax of declaring a method. However, the complete syntax of declaring a method is
- modifier - It defines access types whether the method is public, private, and so on. To learn more, visit Java Access Specifier .
- static - If we use the static keyword, it can be accessed without creating objects. For example, the sqrt() method of standard Math class is static. Hence, we can directly call Math.sqrt() without creating an instance of Math class.
- parameter1/parameter2 - These are values passed to a method. We can pass any number of arguments to a method.
Calling a Method in Java
In the above example, we have declared a method named addNumbers() . Now, to use the method, we need to call it.
Here's is how we can call the addNumbers() method.
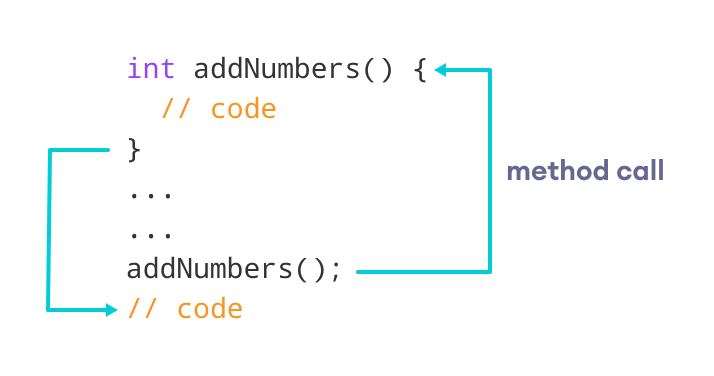
Example 1: Java Methods
In the above example, we have created a method named addNumbers() . The method takes two parameters a and b . Notice the line,
Here, we have called the method by passing two arguments num1 and num2 . Since the method is returning some value, we have stored the value in the result variable.
Note : The method is not static. Hence, we are calling the method using the object of the class.
- Java Method Return Type
A Java method may or may not return a value to the function call. We use the return statement to return any value. For example,
Here, we are returning the variable sum . Since the return type of the function is int . The sum variable should be of int type. Otherwise, it will generate an error.
Example 2: Method Return Type
In the above program, we have created a method named square() . The method takes a number as its parameter and returns the square of the number.
Here, we have mentioned the return type of the method as int . Hence, the method should always return an integer value.
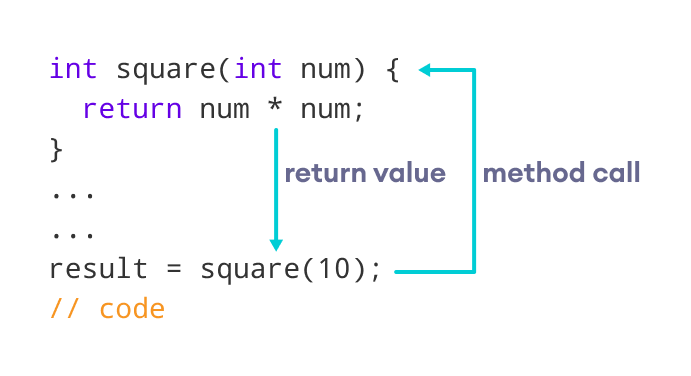
Note : If the method does not return any value, we use the void keyword as the return type of the method. For example,
Method Parameters in Java
A method parameter is a value accepted by the method. As mentioned earlier, a method can also have any number of parameters. For example,
If a method is created with parameters, we need to pass the corresponding values while calling the method. For example,
Example 3: Method Parameters
Here, the parameter of the method is int . Hence, if we pass any other data type instead of int , the compiler will throw an error. It is because Java is a strongly typed language.
Note : The argument 24 passed to the display2() method during the method call is called the actual argument.
The parameter num accepted by the method definition is known as a formal argument. We need to specify the type of formal arguments. And, the type of actual arguments and formal arguments should always match.
- Standard Library Methods
The standard library methods are built-in methods in Java that are readily available for use. These standard libraries come along with the Java Class Library (JCL) in a Java archive (*.jar) file with JVM and JRE.
- print() is a method of java.io.PrintSteam . The print("...") method prints the string inside quotation marks.
- sqrt() is a method of Math class. It returns the square root of a number.
Here's a working example:
Example 4: Java Standard Library Method
To learn more about standard library methods, visit Java Library Methods .
What are the advantages of using methods?
1. The main advantage is code reusability . We can write a method once, and use it multiple times. We do not have to rewrite the entire code each time. Think of it as, "write once, reuse multiple times".
Example 5: Java Method for Code Reusability
In the above program, we have created the method named getSquare() to calculate the square of a number. Here, the method is used to calculate the square of numbers less than 6 .
Hence, the same method is used again and again.
2. Methods make code more readable and easier to debug. Here, the getSquare() method keeps the code to compute the square in a block. Hence, makes it more readable.
Table of Contents
- Calling a Java Method
- Method Parameters
- Advantages of Java Methods
Sorry about that.
Related Tutorials
Java Library
Methods in Java – Explained with Code Examples
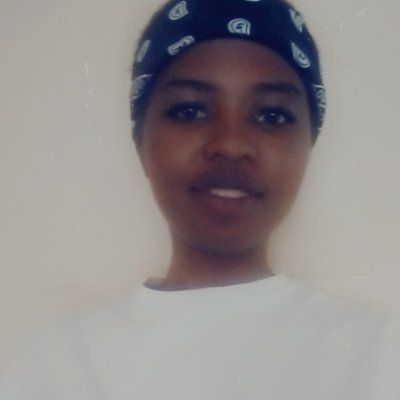
Methods are essential for organizing Java projects, encouraging code reuse, and improving overall code structure.
In this article, we will look at what Java methods are and how they work, including their syntax, types, and examples.
Here's what we'll cover:
What are java methods.
- Types of Access Specifiers in Java – Public ( public ) – Private ( private ) – Protected ( protected ) – Default ( Package-Private )
- Types of Methods – Pre-defined vs. User-defined – Based on functionality
In Java, a method is a set of statements that perform a certain action and are declared within a class.
Here's the fundamental syntax for a Java method:
Let's break down the components:
- accessSpecifier : defines the visibility or accessibility of classes, methods, and fields within a program.
- returnType : the data type of the value that the method returns. If the method does not return any value, the void keyword is used.
- methodName : the name of the method, following Java naming conventions.
- parameter : input value that the method accepts. These are optional, and a method can have zero or more parameters. Each parameter is declared with its data type and a name.
- method body : the set of statements enclosed in curly braces {} that define the task the method performs.
- return statement : if the method has a return type other than void , it must include a return statement followed by the value to be returned.
Here's an example of a simple Java method:
In this example, the addNumbers method takes two integers as parameters ( a and b ), calculates their sum, and returns the result. The main method then calls this method and prints the result.
Compile the Java code using the terminal, using the javac command:

Methods facilitate code reusability by encapsulating functionality in a single block. You can call that block from different parts of your program, avoiding code duplication and promoting maintainability.
Types of Access Specifiers in Java
Access specifiers control the visibility and accessibility of class members (fields, methods, and nested classes).
There are typically four main types of access specifiers: public, private, protected, and default. They dictate where and how these members can be accessed, promoting encapsulation and modularity.
Public ( public )
This grants access to the member from anywhere in your program, regardless of package or class. It's suitable for widely used components like utility functions or constants.
In this example:
- The MyClass class is declared with the public modifier, making it accessible from any other class.
- The publicField is a public field that can be accessed from outside the class.
- The publicMethod() is a public method that can be called from outside the class.
- The main method is the entry point of the program, where an object of MyClass is created, and the public field and method are accessed.
Private ( private )
This confines access to the member within the class where it's declared. It protects sensitive data and enforces encapsulation.
- The MyClass class has a privateField and a privateMethod , both marked with the private modifier.
- The accessPrivateMembers() method is a public method that can be called from outside the class. It provides access to the private field and calls the private method.
Protected ( protected )
The protected access specifier is used to make members (fields and methods) accessible within the same package or by subclasses, regardless of the package. They are not accessible from unrelated classes. It facilitates inheritance while controlling access to specific members in subclasses.
- The Animal class has a protected field ( species ) and a protected method ( makeSound ).
- The Dog class is a subclass of Animal , and it can access the protected members from the superclass.
- The displayInfo() method in the Dog class accesses the protected field and calls the protected method.

With the protected access specifier, members are accessible within the same package and by subclasses, promoting a certain level of visibility and inheritance while still maintaining encapsulation.
Default ( Package-Private )
If no access specifier is used, the default access level is package-private . Members with default access are accessible within the same package, but not outside it. It's often used for utility classes or helper methods within a specific module.
- The Animal class does not have any access modifier specified, making it default (package-private). It has a package-private field species and a package-private method makeSound .
- The Dog class is in the same package as Animal , so it can access the default (package-private) members of the Animal class.
- The Main class runs the program by creating an object of Dog and calling its displayInfo method.
When you run this program, it should output the species and the sound of the animal.
How to Choose the Right Access Specifier
- Public: Use for widely used components, interfaces, and base classes.
- Private: Use for internal implementation details and sensitive data protection.
- Default: Use for helper methods or components specific to a package.
- Protected: Use for shared functionality among subclasses, while restricting access from outside the inheritance hierarchy.
Types of Methods
In Java, methods can be categorized in two main ways:
1. Predefined vs. User-defined:
Predefined methods: These methods are already defined in the Java Class Library and can be used directly without any declaration.
Examples include System.out.println() for printing to the console and Math.max() for finding the maximum of two numbers.
User-defined methods: These are methods that you write yourself to perform specific tasks within your program. They are defined within classes and are typically used to encapsulate functionality and improve code reusability.
- add is a user-defined method because it's created by the user (programmer).
- The method takes two parameters ( num1 and num2 ) and returns their sum.
- The main method calls the add method with specific values, demonstrating the customized functionality provided by the user.
2. Based on functionality:
Within user-defined methods, there are several other classifications based on their characteristics:
Instance Methods:
Associated with an instance of a class. They can access instance variables and are called on an object of the class.
Here are some key characteristics of instance methods:
Access to Instance Variables:
- Instance methods have access to instance variables (also known as fields or properties) of the class.
- They can manipulate the state of the object they belong to.
Use of this Keyword:
- Inside an instance method, the this keyword refers to the current instance of the class. It's often used to differentiate between instance variables and parameters with the same name.
Non-static Context:
- Instance methods are called in the context of an object. They can't be called without creating an instance of the class.
Declaration and Invocation:
- Instance methods are declared without the static keyword.
- They are invoked on an instance of the class using the dot ( . ) notation.
Here's a simple example in Java to illustrate instance methods:
- bark and ageOneYear are instance methods of the Dog class.
- They are invoked on instances of the Dog class ( myDog and anotherDog ).
- These methods can access and manipulate the instance variables ( name and age ) of the respective objects.
Instance methods are powerful because they allow you to encapsulate behavior related to an object's state and provide a way to interact with and modify that state.
Static Methods:
A static method belongs to the class rather than an instance of the class. This means you can call a static method without creating an instance (object) of the class. It's declared using the static keyword.
Static methods are commonly used for utility functions that don't depend on the state of an object. For example, methods for mathematical calculations, string manipulations, and so on.
Abstract Methods:
These methods are declared but not implemented in a class. They are meant to be overridden by subclasses, providing a blueprint for specific functionality that must be implemented in each subclass.
Abstract methods are useful when you want to define a template in a base class or interface, leaving the specific implementation to the subclasses. Abstract methods define a contract that the subclasses must follow.
Other method types: Additionally, there are less common types like constructors used for object initialization, accessor methods (getters) for retrieving object data, and mutator methods (setters) for modifying object data.
In this article, we will look at Java methods, including their syntax, types, and recommended practices.
Happy Coding!
A curious full-stack web developer. I love solving problems using software development and representing Data in a meaningful way. I like pushing myself and taking up new challenges.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Defining Methods
Here is an example of a typical method declaration:
The only required elements of a method declaration are the method's return type, name, a pair of parentheses, () , and a body between braces, {} .
More generally, method declarations have six components, in order:
- Modifierssuch as public , private , and others you will learn about later.
- The return typethe data type of the value returned by the method, or void if the method does not return a value.
- The method namethe rules for field names apply to method names as well, but the convention is a little different.
- The parameter list in parenthesisa comma-delimited list of input parameters, preceded by their data types, enclosed by parentheses, () . If there are no parameters, you must use empty parentheses.
- An exception listto be discussed later.
- The method body, enclosed between bracesthe method's code, including the declaration of local variables, goes here.
Modifiers, return types, and parameters will be discussed later in this lesson. Exceptions are discussed in a later lesson.
The signature of the method declared above is:

Naming a Method
Although a method name can be any legal identifier, code conventions restrict method names. By convention, method names should be a verb in lowercase or a multi-word name that begins with a verb in lowercase, followed by adjectives, nouns, etc. In multi-word names, the first letter of each of the second and following words should be capitalized. Here are some examples:
Typically, a method has a unique name within its class. However, a method might have the same name as other methods due to method overloading .
Overloading Methods
The Java programming language supports overloading methods, and Java can distinguish between methods with different method signatures . This means that methods within a class can have the same name if they have different parameter lists (there are some qualifications to this that will be discussed in the lesson titled "Interfaces and Inheritance").
Suppose that you have a class that can use calligraphy to draw various types of data (strings, integers, and so on) and that contains a method for drawing each data type. It is cumbersome to use a new name for each methodfor example, drawString , drawInteger , drawFloat , and so on. In the Java programming language, you can use the same name for all the drawing methods but pass a different argument list to each method. Thus, the data drawing class might declare four methods named draw , each of which has a different parameter list.
Overloaded methods are differentiated by the number and the type of the arguments passed into the method. In the code sample, draw(String s) and draw(int i) are distinct and unique methods because they require different argument types.
You cannot declare more than one method with the same name and the same number and type of arguments, because the compiler cannot tell them apart.
The compiler does not consider return type when differentiating methods, so you cannot declare two methods with the same signature even if they have a different return type.
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
Methods in Java
This post is targeted to introduce you to methods in Java. By the end of this article, you'll not only know what a method is, but you will be able to understand its Java syntax, why we need it and how you can write a method for your requirements.
What is a method?
A method is composed of a certain number of lines of code, written to perform a specific operation.
For example, you can write a method to get the sum of two numbers. Similarly, when you print a line on the console, the line System.out.println(x); is a method provided by Java to print content. In Java syntax this command means, you want to print x on screen, using the println() method.
Why do we need to use methods?
If you are supposed to divide two numbers, you can write a snippet of code to divide them.
Let's say, you are given to divide two numbers 100 times. Then before writing that snippet 100 times, you might get frustrated by even the thought of it.
Instead of writing redundant lines, if we can write a block of code that you can re-use 100 times. How would that sound? It will not only save us a lot of time, but it will also reduce the memory consumed, increase code reusability, divide your program into small independent units ( modularity ) and lead you to a more sophisticated approach.
How can I write my first method?
Continuing the example of dividing two numbers, let's see how you can write a method to divide two numbers. And how you can re-use it for various numbers.
Explanation
public static int getQuotient(int dividend, int divisor) {
- public is an access modifier. It means you can access this method (referred to as function sometimes) outside the class MethodsInJava too.
- The keyword static notifies that the method getQuotient can be called even without creating an object of class MethodsInJava . This is a concept related to object-oriented programming (you can skip it for now).
- int is the return type of the method. This means, after executing the lines of code, you can return an instance of any data type if you want to. Or keep it void if not required. In this case, the product is returned of type int.
- getQuotient the name of the method.
- (int dividend, int divisor) are parameters passed to the method, of type int . You can pass multiple parameters to a method according to your requirements.
- { This curly brace marks the beginning of the method.
- return dividend / divisor; } returns the quotient by dividing the given parameters.
- } marks the end of the method.
- main() is the driver function where you can call the method getQuotient(dividend, divisor) multiple times by-passing any two integers of your choice. You can write the same method for other data types like double, long etc too.
Print multiples of any integer
Let's look into another example of writing a method to find multiples of a number.
By now you should be clear that we aim to put one complete functionality in one method. After writing your methods you should test them thoroughly for your desired outputs. Or else, if you have consumed them in other functions too, they will impact all the results. So we advise you to practise this concept thoroughly and come back to this article whenever you get stuck. Good luck and happy coding! :)

Hey, it's Tom Bezlar, a pro writer with a CS Master's Degree. I'm a fan of coding, so if you're about to learn Java, stay tuned! I write about tools for Java programming, useful sources for learning to code, tech news, and IT trends general.
- Entrepreneur
- Productivity
- Course List
- First Steps
- Introduction to Java
- Environment setup
- Workspace & First app
- Basic Syntax
- Variables & Constants
- Control Flow
- if, else-if, else, switch
- for, foreach, while, do-while loops
- Intermediate
- Object-oriented Programming
- OOP: Classes & Objects
- OOP: Methods
- OOP: Inheritance
- OOP: Composition
- OOP: Polymorphism
- OOP: Encapsulation
- Lambda Expressions
- Enums (Enumerations)
- List Collections
- ArrayList Collection
- Map Collections
- HashMap Collection
- Errors & Exceptions
- Error & Exception Handling
- Concurrency & Multi-threading
Java OOP: Class Methods Tutorial
In this Java tutorial we learn how to group sections of program functionality into smaller reusable units called methods.
We cover how to define and use non-static and static methods, how to add parameters and how to return values, along with control, from them. We also cover the 'this' construct and how it refers to the calling object as well as how to construct objects with initial values.
Lastly, we quickly discuss and link to the Lambdas lesson and do a quick little challenge.
- What is a method
How to define a method in Java
How to use (invoke/call) a method in java, how to add parameters to a method in java, how to return a value from a method in java, static methods in java, lambda expressions (anonymous methods) in java.
- Mini challenge
The 'this' construct in Java
How to construct an object with values in java.
- Summary: Points to remember
Methods allow us to group sections of our code into a smaller, reusable units.
As an example, let’s consider some simple logic.
The simple logic in the example above will check if a number is even or odd.
Our application may require this evaluation multiple times throughout the code. We don’t want to repeat ourselves and retype this logic each time we want to check a number.
Instead, we want to separate the logic into a unit that we can more easily use over and over, anywhere in our code. These units are called methods.
A method definition consists of the following parts.
- An access modifier
- A return type
- Parameters (optional)
- A body with one or more statements
- A returned value (optional)
In Java, methods can only be defined in classes, we cannot define standalone methods.
We’ll start with the most basic method and add features as we go.
In the example above, we create a class called ‘Logger’ with a method inside called ‘logMessage’. All it does is print some text to the console.
Typically we would create each class in its own file, but to keep things simple, we just defined the ‘Logger’ class below the ‘Program’ class.
Let’s break down the method definition step by step.
- For the access modifier, we used public to allow access to the function from any other class. We cover access modifiers in detail in the OOP: Encapsulation & Access Modifiers lesson .
- For the return type, we used void. When a function doesn’t return a value, we always specify the return type as void.
- Our method has no parameters so we left the parameter list empty. The parentheses must always be included even if the method doesn’t have any parameters.
- In the function body we just print some text to the console.
- Because our function doesn’t return anything, we can omit the return keyword.
Now if we go ahead and run the script, nothing happens. That’s because we’ve defined the method, but we haven’t used anywhere it yet.
To use a method, we need to tell the compiler that we want the method to execute the code in its body once. We do this by invoking the method, otherwise known as calling it.
To call a method we write its name, its parameter list and terminate the statement with a semicolon.
But, because the method is inside a class, we need to instantiate an object and call the method through dot notation. Like we did with parameters.
This time, when we compile and run the script, the text is printed to the console.
Even though we don’t have any parameters, and therefore don’t need any arguments, we still need to add the parentheses in the both the definition and the call.
At this point our method isn’t very useful, it only prints predefined text to the console. With parameters we can change it so that the method prints any message we want.
A parameter is simply a temporary variable that we can use inside the body of the method. The compiler will replace every occurance of the parameter in the definition, with whatever we specify as an argument in the call.
We’ll use our simple log method again.
This time, we’ve added the parameter ‘msg’ of type String in the method’s parameter list. Then, we referred to ‘msg’ where we wanted the value of ‘msg’ to go in the body.
In the method call, we specify the words some text as the argument. Every instance of the ‘msg’ parameter in the body will be replaced by whatever we type here.
From the output we can see that the method printed the text we typed as an argument.
We can also call the method as many times as we want with different messages.
As an example, let’s call the method a few more times with different messages as the argument.
When we run the example above, all the messages are printed to the screen, even the one with an empty string.
Java also allows us to add multiple parameters to our methods. If we have multiple parameters, we separate them with commas, both in the definition and the call.
Methods can return a value to the caller when we invoke them.
As an example, let’s consider a simple math method that adds two numbers together. We may not want to print that value to the page directly from inside the method, but use it for other calculations somewhere else in our application.
We can return the value from the method and either use it directly, or store it in a data container for later use.
To return a value, we write the return keyword, followed by the value we want to return and a semicolon to terminate the statement. We also have to specify the type of value returned in front of the method name in the definition.
The example above will add ‘num2’ to ‘num1’, and return the result when the method is called.
We call the method twice here to demonstrate that it can be used directly in something like the println() method, or we can assign the returned value to a variable to store it.
A static class method is a method that belongs to the class itself, not the instance object of a class.
That means we don’t need an object to call a static class method. We call them directly from the class itself.
To mark a method as static, we simply add the static keyword between the access modifier and the return type.
Now that the method has been marked as static, we can use it without the need to instantiate a class object first.
In the example above, we invoke the method directly by using the class name instead of an object with dot notation.
Lambda expressions always have to be assigned to a reference type of Functional Interfaces.
We cover lambda expressions in the OOP: Lambda Expressions lesson , after we learn about interfaces.
Now that we know more about methods, try to convert the following logic (from the start of the tutorial) into a method.
If you get stuck, see our solutions below.
The this construct is used inside a class to refer to the object that is currently calling it.
As an example, let’s consider the following class.
The ‘greeting’ method’s purpose is to greet a person by their name. When we call the method, it will print ‘Hi, my name is John’ to the console.
The method is doing what it’s supposed to, provided that the programmer passed the correct name as an argument.
If the programmer were to make a mistake and enter something else as the value, the method would no longer function as intended.
In the example above, the method will print the name Jane, instead of John, which is not what we want.
To fix this problem, Java provides us with the this construct, which is used to refer to the object that’s calling the method.
To use the construct, we write the keyword this , followed by the dot operator and a class member name.
We replaced the ‘userName’ parameter in the method with this.name and when we run the example above, it will print the correct name each time the method is called.
Essentially, what’s happening is that Java is replacing the ‘this’ keyword with whatever the object’s name is.
The programmer can no longer make a mistake by entering the wrong name. The compiler will always use the name that’s been assigned to the object.
It makes our code shorter, easier to read and less prone to errors and vulnerabilities.
When we create an instance object of a class, we usualy need to set the attribute values to custom values before we can effectively use them.
We can create and initialize an object in one step with a constructor method. A constructor method will construct the object by assigning values to the attributes when the object is created.
A constructor method has the same name as the class it’s in and doesn’t include a return type.
When an object is created from the class, the constructor method will run.
Inside the constructor method we can initialize properties with values.
In the example above, we specify that the ‘name’ property should be initialized with John whenever we create a new object.
Just like a regular method, Java allows us to accept parameters in the constructor method.
If a constructor has parameters, we have to pass arguments to it when the object is created, otherwise the compiler will raise an error that the constructor is undefined.
- Methods group our logic code into smaller reusable units, and are usually responsible for only a single specific task.
- It’s not enough for a method to be defined, we must call (invoke) it in order to use it.
- Methods can accept one or more parameters that allow different inputs to be used on each call of the method.
- Methods can return a single value with the return keyword.
- We do not need an instance of a class to invoke a static method.
- Java doesn’t require us to use it, but it’s considered good practice to always be as explicit as possible to help avoid errors and vulnerabilities.
- A constructor method has the same name as the class, no return type and may accept parameters.
- A constructor method is automatically called when a new object is created.
- Define a method
- Invoke a method
- Method parameters
- Return from a method
- Static methods
- Lambda expressions
- This calling object
- Constructor method
How to use methods in Java
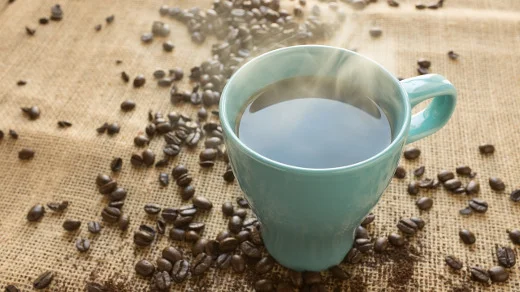
Pixabay. CC0.
A method in Java (called a "function" in many other programming languages) is a portion of code that's been grouped together and labeled for reuse. Methods are useful because they allow you to perform the same action or series of actions without rewriting the same code, which not only means less work for you, it means less code to maintain and debug when something goes wrong.
A method exists within a class, so the standard Java boilerplate code applies:
A package definition isn't strictly necessary in a simple one-file application like this, but it's a good habit to get into, and most IDEs enforce it.
By default, Java looks for a main method to run in a class. Methods can be made public or private, and static or non-static, but the main method must be public and static for the Java compiler to recognize and utilize it. When a method is public, it's able to be executed from outside the class. To call the Example class upon start of the program, its main method must be accessible, so set it to public .
Here's a simple demonstration of two methods: one main method that gets executed by default when the Example class is invoked, and one report method that accepts input from main and performs a simple action.
To mimic arbitrary data input, I use an if-then statement that chooses between two strings, based on when you happen to start the application. In other words, the main method first sets up some data (in real life, this data could be from user input, or from some other method elsewhere in the application), and then "calls" the report method, providing the processed data as input:
Run the code:
Notice that there are two different results from the same report method. In this simple demonstration, of course, there's no need for a second method. The same result could have been generated from the if-then statement that mimics the data generation. But when a method performs a complex task, like resizing an image into a thumbnail and then generating a widget on screen using that resized image, then the "expense" of an additional component makes a lot of sense.
When to use a Java method
It can be difficult to know when to use a method and when to just send data into a Java Stream or loop. If you're faced with that decision, the answer is usually to use a method. Here's why:
- Methods are cheap. They don't add processing overhead to your code.
- Methods reduce the line count of your code.
- Methods are specific. It's usually easier to find a method called resizeImage than it is to find code that's hidden in a loop somewhere in the function that loads images from the drive.
- Methods are reusable. When you first write a method, you may think it's only useful for one task within your application. As your application grows, however, you may find yourself using a method you thought you were "done" with.
Functional vs. object-oriented programming
Functional programming utilizes methods as the primary construct for performing tasks. You create a method that accepts one kind of data, processes that data, and outputs new data. String lots of methods together, and you have a dynamic and capable application. Programming languages like C and Lua are examples of this style of coding.
The other way to think of accomplishing tasks with code is the object-oriented model, which Java uses. In object-oriented programming, methods are components of a template. Instead of sending data from method to method, you create objects with the option to alter them through the use of their methods.
Here's the same simple zombie apocalypse demo program from an object-oriented perspective. In the functional approach, I used one method to generate data and another to perform an action with that data. The object-oriented equivalent is to have a class that represents a work unit. This example application presents a message-of-the-day to the user, announcing that the day brings either a zombie party or a zombie apocalypse. It makes sense to program a "day" object, and then to query that day to learn about its characteristics. As an excuse to demonstrate different aspects of object-oriented construction, the new sample application will also count how many zombies have shown up to the party (or apocalypse).
Java uses one file for each class, so the first file to create is Day.java , which serves as the Day object:
In the Class section, two fields are created: weather and count . Weather is static. Over the course of a day (in this imaginary situation), weather doesn't change. It's either a party or an apocalypse, and it lasts all day. The number of zombies, however, increases over the course of a day.
In the Constructor section, the day's weather is determined. It's done as a constructor because it's meant to only happen once, when the class is initially invoked.
In the Methods section, the report method only returns the weather report as determined and set by the constructor. The counter method, however, generates a random number and adds it to the current zombie count.
This class, in other words, does three very different things:
- Represents a "day" as defined by the application.
- Sets an unchanging weather report for the day.
- Sets an ever-increasing zombie count for the day.
To put all of this to use, create a second file:
Because there are now two files, it's easiest to use a Java IDE to run the code, but if you don't want to use an IDE, you can create your own JAR file . Run the code to see the results:
The "weather" stays the same regardless of how many times the report method is called, but the number of zombies on the loose increases the more you call the counter method.
Java methods
Methods (or functions) are important constructs in programming. In Java, you can use them either as part of a single class for functional-style coding, or you can use them across classes for object-oriented code. Both styles of coding are different perspectives on solving the same problem, so there's no right or wrong decision. Through trial and error, and after a little experience, you learn which one suits a particular problem best.
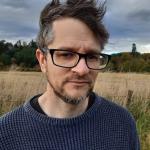
Comments are closed.
Related content.

Writing Methods
As you know, objects have behaviour and that behaviour is implemented by its methods. Other objects can ask an object to do something by invoking its methods. This section tells you everything you need to know to write methods for your Java classes. For more information about how to call methods see Using an Object . In Java, you define a class's methods within the body of the class for which the method implements some behaviour. Typically, you declare a class's methods after its variables in the class body although this is not required. Similar to a class implementation, a method implementation consists of two parts: the method declaration and the method body. methodDeclaration { . . . methodBody . . . }
The Method Declaration
At minimum, the method declaration component of a method implementation has a return type indicating the data type of the value returned by the method, and a name. returnType methodName() { . . . }
class Stack { . . . boolean isEmpty() { . . . } }
The Method Body
The method body is where all of the action of a method takes place; the method body contains all of the legal Java instructions that implement the method. For example, here is the Stack class with an implementation for the isEmpty() method. class Stack { static final int STACK_EMPTY = -1; Object stackelements[]; int topelement = STACK_EMPTY; . . . boolean isEmpty() { if (topelement == STACK_EMPTY) return true; else return false; } }
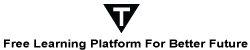
Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- Enterprise Java
- Web-based Java
- Data & Java
- Project Management
- Visual Basic
- Ruby / Rails
- Java Mobile
- Architecture & Design
- Open Source
- Web Services

Developer.com content and product recommendations are editorially independent. We may make money when you click on links to our partners. Learn More .
There are a few guidelines that one must adhere to while writing code that really is clean and understandable. One such simple technique is the single responsibility principle. This article glimpses over six key concepts of writing efficient methods in Java .
Methods, in a way, are the action points in Java. A method typically consists of a set of well-defined program statements. It has a name and a set of different types of arguments (0 or more). The type of processing it does is conventionally conveyed by the name it is referred to. It can be invoked at any point in the program by using its name and arguments only. It literally is a subprogram within the main program. There can be many methods in a program. Each method is distinct due to its name, number, and type of argument it takes. In an Object-Oriented Language such as Java, a method can be extended by the technique called method overloading or polymorphism. A method may or may not return a value as a final statement. The names of the method and the argument are distinctively called its signature. It is a part of method declaration.
1. Method Signature
Although a method may have different return types, it does not add to its distinctiveness. Therefore, it is not included in the ‘method signature’.
Listing 1 : Method with distinct return type does not add to the distinctive need of the method
Note that the methods declared above are only distinct by their return type, which the compiler does not recognize; hence, it flags an error of a repeated declaration. Instead, if we change the arguments in the methods, they all become valid. This type of method with the same name but a different argument list is called method overloading . Note that the Java compiler distinguishes them by their signatures.
Listing 2 : Valid overloaded method
The reason for not including the return type in the method signature is that, as we can see in Listing 1 , there is no way to distinguish between which method is invoked from the following two valid method calls.
It is all right to invoke a method and ignore the result. Therefore, to prohibit ambiguity, the Java compiler does not allow method overloading on the basis of return type. A method signature is a mark of its distinctiveness, so a return type it is not taken into account as its signature.
2. Method Body
A method exists as a definition of an action. This action is explicated through a logical set of program statements. The simplest guideline to express the logic is that it must perform a single, well-defined task in as optimum a manner as possible. The definition should be simple enough to be identified the method name given. This is the single responsibility principle . The method should be reasonably short, crisp, and readable. Apply appropriate comments to enhance the readability as needed. It is better to lay the exit point logic in such a manner that there is only one return statement. For example, a code statement such as,
can be easily converted as follows:
A shorter version may be written as follows, but it limits readability. Therefore, it should be avoided.
This is a bit better.
3. Method Overloading
Method overloading is when we use more than one method with the same name but different argument type, number of arguments, and combination. The Java compiler picks the appropriate method on invocation according to the signature. For example, we can find a number of methods overloaded in the String class of Java API, such as:
Returns the index within this string of the first occurrence of the specified character. (Java API Doc)
Returns the index within this string of the first occurrence of the specified character, starting the search at the specified index. (Java API Doc)
Returns the index within this string of the first occurrence of the specified substring. (Java API Doc)
Method overloading may remind you of generics in Java but note that they are not the same. They serve different purposes. We’ll pick them up in separate article because they need more explanation. Nevertheless, they can go side by side and are a pretty powerful combination.
4. Method Overriding
Method overriding is where a method is inherited from a parent class by the child class to extend its implementation to convey a new meaning. It is significantly different from method overloading in the sense that here the method name, type, and number of arguments must remain the same all throughout the inheritance. We typically use the @Override annotation to emphasize that we intend to override the parent method and not overload it.
The Java compiler flags an error if we, by any chance, make any mistake in the method signature. This is the reason the @Override annotation is very important.
5. Recursion
A method that calls itself to perform a calculation is called a recursive method . Recursive logic often makes the implementation logic terse and crisp. There is no such recursive logic that cannot be implemented with simple loops. But, the main use of recursive implementation is to make the program logic short and simple. There is some specific calculation which can be better defined through recursive logic, such as implementing Tower of Hanoi, GCD, factorial, and so forth. It is better to avoid unnecessary recursion because it may obfuscate code to the verge of making it hard to debug and read. Recursion heavily uses a system stack and, based upon the stack size, the JVM may throw a StackOverflowError exception at runtime. It is unlikely, yet a probable situation depending upon how deep the call chain goes.
6. Method Documentation
Although Java never enforces one, methods should be documented by using the Javadoc tool. This is particularly useful if we are developing some sort of a library or a framework. A well-documented code helps other programmers to understand the purpose of the method, number, and type of argument it takes and the constraints associated with the implementation. It also gives an idea about the exception it may raise and the value it returns, if any.
The preceding code is an extract from the Java API source to illustrate verbosity. Popular Java IDEs, like Eclipse and IntelliJ Idea, pick up the documentation to provide useful information while coding. This immensely helps programmers at all levels to get a precise idea about the purpose and syntax of the method quickly.
These six vital but not comprehensive points should be adhered to in writing efficient methods in Java. A code that does not enhance readability is not a good code, however cleverly it may be implemented. Remember that efficiency lies not in employing tricky code but on simplicity. It is good idea to divide a complicated logic into more than make one big method. Try debugging such code you’ll understand what it takes. The key idea is to apply the KISS principle as far as possible.
Get the Free Newsletter!
Subscribe to Developer Insider for top news, trends & analysis
Latest Posts
What is the role of a project manager in software development, how to use optional in java, overview of the jad methodology, microsoft project tips and tricks, how to become a project manager in 2023, related stories.
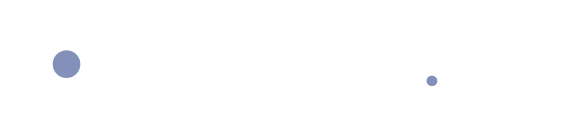
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
Java provides some pre-defined methods, such as System.out.println(), but you can also create your own methods to perform certain actions: Example. ... Call a Method. To call a method in Java, write the method's name followed by two parentheses and a semicolon; In the following example, ...
Declaring a Java Method. The syntax to declare a method is: returnType methodName() { // method body } Here, returnType - It specifies what type of value a method returns For example if a method has an int return type then it returns an integer value. If the method does not return a value, its return type is void.; methodName - It is an identifier that is used to refer to the particular method ...
The method in Java or Methods of Java is a collection of statements that perform some specific tasks and return the result to the caller. A Java method can perform some specific tasks without returning anything. Java Methods allows us to reuse the code without retyping the code. In Java, every method must be part of some class that is different from languages like C, C++, and Python.
Simple Java method. In this example, the addNumbers method takes two integers as parameters ( a and b ), calculates their sum, and returns the result. The main method then calls this method and prints the result. Compile the Java code using the terminal, using the javac command: Output.
Defining Methods. Here is an example of a typical method declaration: double length, double grossTons) {. //do the calculation here. The only required elements of a method declaration are the method's return type, name, a pair of parentheses, (), and a body between braces, {}. More generally, method declarations have six components, in order:
A method is a block of code or collection of statements or a set of code grouped together to perform a certain task or operation. It is used to achieve the reusability of code. We write a method once and use it many times. We do not require to write code again and again.
In Java, methods are where we define the business logic of an application. They define the interactions among the data enclosed in an object. In this tutorial, we'll go through the syntax of Java methods, the definition of the method signature, and how to call and overload methods. 2. Method Syntax.
A method is composed of a certain number of lines of code, written to perform a specific operation. For example, you can write a method to get the sum of two numbers. Similarly, when you print a line on the console, the line System.out.println(x); is a method provided by Java to print content.
2. Method Bodies. The code within each method, also known as method bodies, is written for certain tasks to be performed. It is executed when the method is called and consists of the simplest of ...
To call a method we write its name, its parameter list and terminate the statement with a semicolon. But, because the method is inside a class, we need to instantiate an object and call the method through dot notation. ... Just like a regular method, Java allows us to accept parameters in the constructor method. Example: Copy. public class ...
Methods can be made public or private, and static or non-static, but the main method must be public and static for the Java compiler to recognize and utilize it. When a method is public, it's able to be executed from outside the class. To call the Example class upon start of the program, its main method must be accessible, so set it to public.
Method header = modifier+return type + method name + parameter list. Method signature = method name + parameter list. We can simplify the method structure further like this. Figure 2 — A ...
click here. Method 2 (Using local classes) You can also implement a method inside a local class. A class created inside a method is called local inner class. If you want to invoke the methods of local inner class, you must instantiate this class inside method. Method 3 (Using a lambda expression)
In Java, you define a class's methods within the body of the class for which the method implements some behaviour. Typically, you declare a class's methods after its variables in the class body although this is not required. Similar to a class implementation, a method implementation consists of two parts: the method declaration and the method body.
Let's check some different ways to write the main method. Although they're not very common, they're valid signatures. Note that none of these are specific to the main method, they can be used with any Java method but they are also a valid part of the main method.. The square brackets can be placed near String, as in the common template, or near args on either side:
The method definition consists of a method header and method body. We can call a method by using the following: method_name (); //non static method calling. If the method is a static method, we use the following: obj.method_name (); //static method calling. Where obj is the object of the class.
This article glimpses over six key concepts of writing efficient methods in Java. Overview. Methods, in a way, are the action points in Java. A method typically consists of a set of well-defined program statements. It has a name and a set of different types of arguments (0 or more). The type of processing it does is conventionally conveyed by ...
Conclusion. Java Generics is a powerful addition to the Java language because it makes the programmer's job easier and less error-prone. Generics enforce type correctness at compile time and, most importantly, enable the implementation of generic algorithms without causing any extra overhead to our applications.
1. To formats decimal numbers, you can use java.text.DecimalFormat, define it in the class level as a class member so that it could share it's behavior for all objects of Payroll as @Multithreader mentioned it in the comment, this will be your toString() method: private static DecimalFormat df = new DecimalFormat("#.##");
The toString() method returns a textual representation of an object. A basic implementation is already included in java.lang.Object and so because all objects inherit from java.lang.Object it is guaranteed that every object in Java has this method. Overriding the method is always a good idea, especially when it comes to debugging, because ...
Syntax to call a static method: className.methodName(); Example 1: The static method does not have access to the instance variable. The JVM runs the static method first, followed by the creation of class instances. Because no objects are accessible when the static method is used. A static method does not have access to instance variables.
I am trying to use Mockito and PowerMockito to write a Test case for the first time, but I am not able to run it successfully. I am having trouble mocking a method "delete" present in class HbaseDaoImpl, which is available as an Instance variable in class HbaseIngestionBase, and Class HbaseIngestionBase is being extended by class DeleteDataFromHBase.
48 likes, 0 comments - amitguptaazone on May 7, 2024: "Day 13/100 / Today I practice Questions on methods 1- Write a Java method to find the smallest number among ...